📩 How to Integrate an SMS Sending Service in Laravel using notify.lk SMS Gateway.
In this tutorial, you’ll learn how to integrate a custom SMS sending service into your Laravel application. We’ll move an the sms
folder to app/Services
, create a reusable SmsService
class, and use it within a new SmsController
. Finally, we’ll set up a route to test the SMS functionality via a browser. By the end, your Laravel app will have a clean structure with modular SMS logic, ready for production use.
Download Code : https://github.com/notifylk/notify-php/blob/master/README.md
1. Place the sms
Folder in app/Services
Move your sms
folder (with autoload.php
) to the app/Services
directory in your Laravel project.
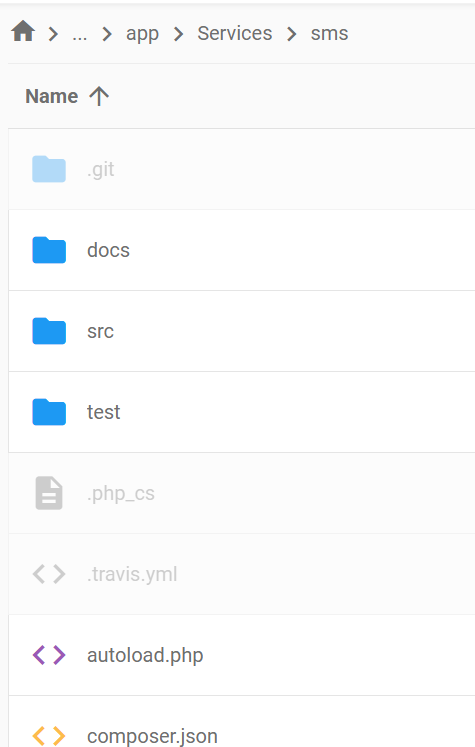
2. Create the SmsService
Class
This class will encapsulate the SMS sending logic.
app/Services/SmsService.php
<?php
namespace App\Services;
require_once base_path('app/Services/sms/autoload.php');
use NotifyLk\Api\SmsApi;
class SmsService
{
protected $api_instance;
public function __construct()
{
$this->api_instance = new SmsApi();
}
public function sendSMS($user_id, $api_key, $message, $to, $sender_id)
{
try {
$response = $this->api_instance->sendSMS(
$user_id,
$api_key,
$message,
$to,
$sender_id
);
return $response;
} catch (\Exception $e) {
return 'Exception when calling SmsApi->sendSMS: ' . $e->getMessage();
}
}
}
3. Create the SmsController
This controller will use the SmsService
to send an SMS.
app/Http/Controllers/SmsController.phpv1
<?php
namespace App\Http\Controllers;
use App\Services\SmsService;
use App\Models\Contributor;
use Illuminate\Support\Carbon;
class SmsController extends Controller
{
public function send()
{
$targetDate = Carbon::now()->addDays(2)->toDateString();
// Query contributors where any date matches target date
$contributors = \App\Models\Contributor::where(function($query) use ($targetDate) {
$query->whereDate('date', $targetDate)
->orWhereDate('date_2', $targetDate)
->orWhereDate('date_3', $targetDate)
->orWhereDate('date_4', $targetDate);
})
->whereNotNull('mobile')
->where('mobile', '!=', '')
->get();
$smsService = new SmsService();
$response = "";
foreach ($contributors as $contributor) {
// Example: Determine the matching date field
$almsgivingDate = null;
if ($contributor->date == $targetDate) {
$almsgivingDate = $contributor->date;
} elseif ($contributor->date_2 == $targetDate) {
$almsgivingDate = $contributor->date_2;
} elseif ($contributor->date_3 == $targetDate) {
$almsgivingDate = $contributor->date_3;
} elseif ($contributor->date_4 == $targetDate) {
$almsgivingDate = $contributor->date_4;
}
$alms = null;
if ($contributor->alms != "") {
$alms = ($contributor->alms == 0) ? "Heel" : "Dawal";
} elseif ($contributor->alms_2 != "") {
$alms = ($contributor->alms_2 == 0) ? "Heel" : "Dawal";
} elseif ($contributor->alms_3 != "") {
$alms = ($contributor->alms_3 == 0) ? "Heel" : "Dawal";
} elseif ($contributor->alms_4 != "") {
$alms = ($contributor->alms_4 == 0) ? "Heel" : "Dawal";
}
// Remove spaces
$mobile = str_replace(' ', '', $contributor->mobile);
// Remove first 0 if present
if (substr($mobile, 0, 1) === '0') {
$mobile = substr($mobile, 1);
}
// Add 94 at the beginning
$mobile = '94' . $mobile;
// Ensure the total length is 11
if (strlen($mobile) === 11) {
// $mobile is now correctly formatted, e.g. 947XXXXXXXX
} else {
// Handle invalid mobile number (log, skip, etc.)
}
$user_id = "123456"; // Replace with your API User ID
$api_key = "ahdsahdadjadadha234672462424"; // Replace with your API Key
$message = "{$contributor->name}, Blessings of the Triple Gem! \n\nYour next almsgiving: {$almsgivingDate} ('{$alms}') at Maha Mangala Viharaya, Matugama. \n\nMore details & stop notifications https://temples.japura.lk \n\nThank you!";
$to = $mobile; // Replace with the recipient's phone number
$sender_id = "NotifyDEMO"; // Replace with your sender ID
$contact_fname = "dsd"; // string | Contact First Name - This will be used while saving the phone number in your Notify contacts (optional).
$contact_lname = "dsds"; // string | Contact Last Name - This will be used while saving the phone number in your Notify contacts (optional).
$contact_email = "dsds"; // string | Contact Email Address - This will be used while saving the phone number in your Notify contacts (optional).
$contact_address = "dsds"; // string | Contact Physical Address - This will be used while saving the phone number in your Notify contacts (optional).
$contact_group = 0; // int | A group ID to associate the saving contact with (optional).
$type = "unicode"; // Default is null string | Message type. Provide as unicode to support unicode (optional).
$response = $smsService->sendSMS($user_id, $api_key, $message, $to, $sender_id, $contact_fname, $contact_lname, $contact_email, $contact_address, $contact_group, $type);
}
return $response;
}
}
4. Define the Route
Add a route in routes/web.php
to trigger the SMS sending functionality.
routes/web.php
use App\Http\Controllers\SmsController;
Route::get('/send-sms', [SmsController::class, 'send']);
5. Folder Structure
Your Laravel project should now have the following structure:
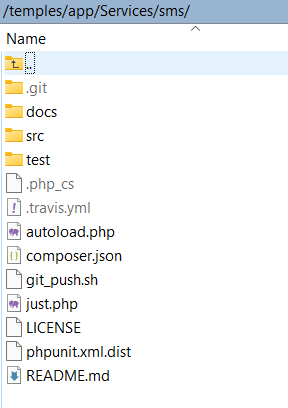
app/
Services/
sms/
autoload.php
SmsService.php
Http/
Controllers/
SmsController.php
routes/
web.php
Code :
<?php
namespace App\Http\Controllers;
use App\Services\SmsService;
class SmsController extends Controller
{
public function send()
{
$smsService = new SmsService();
$user_id = "123456"; // Replace with your API User ID
$api_key = "sadhjadadhasdasd3232323"; // Replace with your API Key
$message = "Hello, this is a test message!";
$to = "94714676522"; // Replace with the recipient's phone number
$sender_id = "NotifyDEMO"; // Replace with your sender ID
$response = $smsService->sendSMS($user_id, $api_key, $message, $to, $sender_id);
return $response;
}
}
<?php
namespace App\Services;
require_once base_path('app/Services/sms/autoload.php');
use NotifyLk\Api\SmsApi;
class SmsService
{
protected $api_instance;
public function __construct()
{
$this->api_instance = new SmsApi();
}
public function sendSMS($user_id, $api_key, $message, $to, $sender_id)
{
try {
$response = $this->api_instance->sendSMS(
$user_id,
$api_key,
$message,
$to,
$sender_id
);
return $response;
} catch (\Exception $e) {
return 'Exception when calling SmsApi->sendSMS: ' . $e->getMessage();
}
}
}